In this article, we learn how to get checkbox values from the form on submit. During the process, we will implement the following functionalities:
- Display all checkbox options on screen.
- Allow users to check and uncheck any option
- Display checked options on submit.
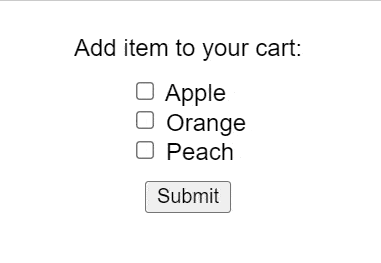
1. Create checkbox for every option
First, we will add HTML code to display the checkboxes with input type “checkbox”. To ensure that all the checkbox comes under the same input name, “cartitems” name is assigned to every checkbox element.
<div>
Add item to your cart:
</div>
<div>
<input type="checkbox" id="item1" name="cartitems" />
<label for="item1">Apple</label><br />
<input type="checkbox" id="item2" name="cartitems" />
<label for="item2">Orange</label><br />
<input type="checkbox" id="item3" name="cartitems" />
<label for="item3">Peach</label>
</div>
2. Assign unique value to each checkbox
Once the checkboxes are created, we need to add unique values for each checkbox to differentiate them.
<input type="checkbox" id="item1" name="cartitems" value="apple" />
<label for="item1">Apple</label><br />
<input type="checkbox" id="item2" name="cartitems" value="orange" />
<label for="item2">Orange</label><br />
<input type="checkbox" id="item3" name="cartitems" value="peach" />
<label for="item3">Peach</label>
3. Enclose <input/> with <form></form> tag
The <form></form> tag provides direct access to all the child <input> elements. Hence, once the checkboxes are enclosed by <form></form>, we will be able to access checkbox values from “document.form[‘user-cart’]“.
<form id="user-cart">
<div id="app">
<div>
Add item to your cart:
</div>
<div>
<input type="checkbox" id="item1" name="cartitems" value="apple" />
<label for="item1">Apple</label><br />
<input type="checkbox" id="item2" name="cartitems" value="orange" />
<label for="item2">Orange</label><br />
<input type="checkbox" id="item3" name="cartitems" value="peach" />
<label for="item3">Peach</label>
</div>
</div>
</form>
4. Button to submit and display the selected checkbox values
Next, we need to create the following HTML elements:
- HTML Button to allow users to submit the checkbox
- <div></div> container where the selected values are displayed.
<button id="submit-cart">Submit</button>
<div id="display-cart"></div>
The HTML elements can be accessed through JavaScript using “document.getElementById” and forms elements through “document.forms“.
var submitCart = document.getElementById("submit-cart");
var userCart = document.forms["user-cart"];
var displayCart = document.getElementById("display-cart");
5. JavaScript Function to get the checked values
The handleSubmit function is triggered on a button click using JavaScript events. In the handleSubmit function, we perform the following tasks:
- Avoid page refresh using “event.preventDefault()“.
- Retrieve list of all checkbox elements using ‘userCart.elements[“cartitems”]‘.
- Filter all unchecked elements through the forEach() loop.
- Display values of all the checked elements on the screen.
// Attach function to handle button click
submitCart.addEventListener("click", handleSubmit);
// Function to display selected value on screen
function handleSubmit(event) {
// Avoid page refresh
event.preventDefault();
var allOptions = userCart.elements["cartitems"];
var selectedOptions = [];
allOptions.forEach((element) => {
if (element.checked) {
selectedOptions.push(element.value);
}
});
// Display the selected checkboxes on screen
var cartString = selectedOptions.join(", ");
displayCart.innerText = `You have selected: ${cartString}`;
}
Final Solution Code
index.html
<!DOCTYPE html>
<html>
<head>
<title>Get selected option value</title>
<link rel="stylesheet" href="styles.css" />
<script src="main.js" defer></script>
</head>
<body>
<form id="user-cart">
<div id="app">
<div>
Add item to your cart:
</div>
<div>
<input type="checkbox" id="item1" name="cartitems" value="apple" />
<label for="item1">Apple</label><br />
<input type="checkbox" id="item2" name="cartitems" value="orange" />
<label for="item2">Orange</label><br />
<input type="checkbox" id="item3" name="cartitems" value="peach" />
<label for="item3">Peach</label>
</div>
<button id="submit-cart">Submit</button>
<div id="display-cart"></div>
</div>
</form>
</body>
</html>
main.js
var submitCart = document.getElementById("submit-cart");
var userCart = document.forms["user-cart"];
var displayCart = document.getElementById("display-cart");
// Attach function to handle button click
submitCart.addEventListener("click", handleSubmit);
// Function to display selected value on screen
function handleSubmit(event) {
// Avoid page refresh
event.preventDefault();
var allOptions = userCart.elements["cartitems"];
var selectedOptions = [];
allOptions.forEach((element) => {
if (element.checked) {
selectedOptions.push(element.value);
}
});
var cartString = selectedOptions.join(", ");
displayCart.innerText = `You have selected: ${cartString}`;
}
styles.css
#app {
font-family: sans-serif;
height: 100vh;
display: flex;
gap: 10px;
justify-content: center;
align-items: center;
flex-direction: column;
}
.display-value {
margin-top: 20px;
}