React JS internally uses virtual DOM over standard JavaScript DOM methods, hence “element.addEventListener()” isn’t ideal for React JS code. Hence, the most effective method to add an onClick event lister is by specifying the handler function in inline JSX code.
In this article, we will demonstrate adding the onClick event by creating React JS app with the following features:
- Allow users to type the name in the input box.
- Submit the input on button click.
- Reset the value on button click.
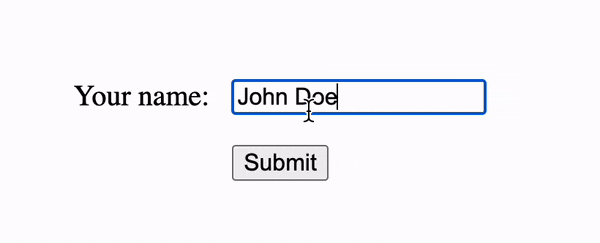
1. Declare React States to manage user input
React States ensure that user input and actions are reflected on the screen. Further, the state values are accessible through the onClick handler function.
- name: State to control name input by the user.
- isReset: Boolean state which indicated if the input has been reset.
const [name, setName] = useState();
const [isReset, setIsReset] = useState(true);
2. <input /> element for parameter values
The input value entered by the user must be set to React state. In the below example, we update the “name” state with “event.target.value” from the input element.
const handleNameChange = (event) => {
setName(event.target.value);
};
The onChange handler ensures that the React state is up to date with the user-entered value.
<div className="user-input">
<label>Your name: </label>
<input id="user-name" name="name" onChange={handleNameChange} />
</div>
3. Create event handler functions
The JavaScript handler function will be executed when the respective onClick event is triggered. Below, we define the onClick handler for both submit and reset buttons.
const handleSubmit = () => {
setIsReset(false);
};
const handleReset = () => {
setName("");
setIsReset(true);
};
4. Assign onClick event to the button element
Specifying event handlers inline is the ideal approach to adding event listeners in React JS. Hence, the “onChange” and “onClick” attributes of HTML elements are assigned with event handler functions.
<div className="user-input">
<label>Your name: </label>
<input id="user-name" name="name" onChange={handleNameChange} />
</div>
<div>
<button id="user-submit" onClick={handleSubmit}>
Submit
</button>
</div>
<div>Hello {name}!</div>
<button id="user-submit" onClick={handleReset}>
Reset
</button>
5. Display the parameters on the screen
React conditional rendering returns JSX code based on the condition, including React state reflects the changes on the screen for each function call of the “setState()“.
Each time the setIsReset() is called, the JSX returned by “isReset ? renderInputName : renderResetName” will be changed based on the updated value.
<div className="main">{isReset ? renderInputName : renderResetName}</div>
Final Solution Code
App.js
import { useState } from "react";
import "./styles.css";
export default function App() {
// React state to manage form elements
const [name, setName] = useState();
const [isReset, setIsReset] = useState(true);
// Update state with user input
const handleNameChange = (event) => {
setName(event.target.value);
};
const handleSubmit = () => {
setIsReset(false);
};
const handleReset = () => {
setName("");
setIsReset(true);
};
// JSX code to accept user input
const renderInputName = (
<>
<div className="user-input">
<label>Your name: </label>
<input id="user-name" name="name" onChange={handleNameChange} />
</div>
<div>
<button id="user-submit" onClick={handleSubmit}>
Submit
</button>
</div>
</>
);
// JSX code to display entered value
const renderResetName = (
<>
<div>Hello {name}!</div>
<button id="user-submit" onClick={handleReset}>
Reset
</button>
</>
);
// Conditional rendering based on isReset State value
return (
<div className="main">{isReset ? renderInputName : renderResetName}</div>
);
}
styles.css
.main {
display: flex;
justify-content: center;
flex-direction: column;
align-items: center;
gap: 20px;
height: 100vh;
font-size: 20px;
}
.user-input {
display: flex;
gap: 15px;
}
#user-submit,
#user-name {
font-size: 16px;
}