In this article, we will learn how to use React.createContext in React JS project and access it across multiple components. We will accomplish the following steps:
- Define context using React.createContext().
- Wrap JSX content with <Context.Provider> </Context.Provider> with context object as value.
- Access context object from child component through useContext().
1. Declare React JS Context using React.createContext()
The first step is to define the React Context instance using createContext() and assign it to a JS variable. The instance can be later used to access context values.
export const MainContext = React.createContext();
2. Define context object with properties and values
JS constant “data” is declared with the object of key-value pairs of parentValue and childValue properties.
const data = {
parentValue: "Parent Body",
childValue: "Child Body"
};
3. Wrapping App component with <Context.Provider> </Context.Provider>
We wrap the content of App component with <Context.Provider> </Context.Provider>, this the context object to be accessed from child component.
// App Component
function App() {
return (
<MainContext.Provider value={data}>
<ParentComponent />
</MainContext.Provider>
);
}
4. useContext() to access context object from child component
The context object is accessed from the child component with useContext() method with the previously defined context instance as a parameter.
const data = useContext(MainContext);
5. Include context values in JSX code of React Component
Finally, we will include the “data.childValue” from the context object in JSX code returned from the Child component.
import React, { useContext } from "react";
import { MainContext } from "./App";
// Nested Child Component
const ChildComponent = () => {
const data = useContext(MainContext);
return <div>{data.childValue}</div>;
};
export default ChildComponent;
Final Solution Code
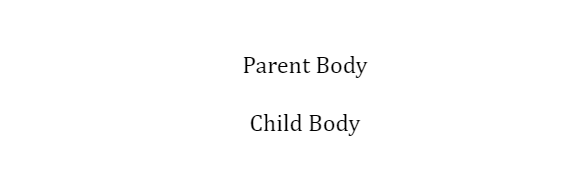
App.js
import React from "react";
import ParentComponent from "./ParentComponent";
import ReactDOM from "react-dom";
import "./styles.css";
export const MainContext = React.createContext();
const data = {
parentValue: "Parent Body",
childValue: "Child Body"
};
// Main Component
function App() {
return (
<MainContext.Provider value={data}>
<ParentComponent />
</MainContext.Provider>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
ParentComponent.js
import React, { useContext } from "react";
import { MainContext } from "./App";
import ChildComponent from "./ChildComponent";
// Child of Main Component
const ParentComponent = () => {
const data = useContext(MainContext);
return (
<div className="app">
{data.parentValue}
<ChildComponent />
</div>
);
};
export default ParentComponent;
ChildComponent.js
import React, { useContext } from "react";
import { MainContext } from "./App";
// Nested Child Component
const ChildComponent = () => {
const data = useContext(MainContext);
return <div>{data.childValue}</div>;
};
export default ChildComponent;
styles.css
.app {
font-family: sans-serif;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
gap: 20px;
height: 100vh;
font-family: Cambria, Cochin, Georgia, Times, "Times New Roman", serif;
}