In this article, we will learn how to build a search filter for the list of items using React JS. The React Search filter will accomplish the following functionalities:
- Search box to allow users to input search query.
- Display filtered list of items.
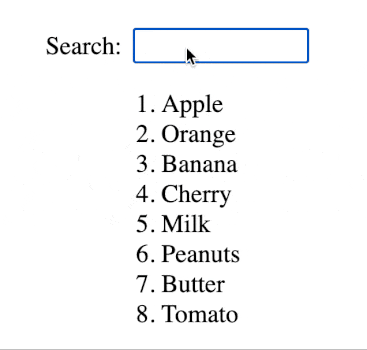
1. Declare the list of items
First, we need to create initialize a JS constant with a list of all items which will be later used in the application.
const itemList = [
"Apple",
"Orange",
"Banana",
"Cherry",
"Milk",
"Peanuts",
"Butter",
"Tomato"
];
2. Add React state for filtered list of items
We will create filteredList React state with itemList constant as the initial value. This allows the item list rendered on the screen to be updated for every setFilteredList() call.
const [filteredList, setFilteredList] = new useState(itemList);
3. Render list of all items on screen
We render the filteredList array using the map() function and return an ordered list for every entry. Read more about JavaScript map() from developer.mozilla.org/JavaScript/Array/map.
return (
<div id="item-list">
<ol>
{filteredList.map((item, index) => (
<li key={index}>{item}</li>
))}
</ol>
</div>
);
4. Function to filter the list by search query
Now we need a function to filter the item list based on the search query. We accomplish the functionality in 4 steps:
- Access the search query value using “event.target.value“.
- Make a copy of list of items.
- Filter list to include items with search query.
- setState() to update the list with filtered values.
const filterBySearch = (event) => {
// Access input value
const query = event.target.value;
// Create copy of item list
var updatedList = [...itemList];
// Include all elements which includes the search query
updatedList = updatedList.filter((item) =>
return item.toLowerCase().indexOf(query.toLowerCase()) !== -1;
});
// Trigger render with updated values
setFilteredList(updatedList);
};
5. Allow users to type seach query
To allows users to type the search query, we need to
- Create HTML input element to allow entry of search query.
- Add filterBySearch() as onChange for input element.
return (
<div className="App">
<div className="search-header">
<div className="search-text">Search:</div>
<input id="search-box" onChange={filterBySearch} />
</div>
<div id="item-list">
<ol>
{filteredList.map((item, index) => (
<li key={index}>{item}</li>
))}
</ol>
</div>
</div>
);
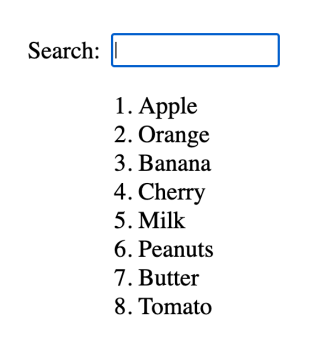
Final Solution Code
App.js
import "./styles.css";
import React, { useState } from "react";
export default function App() {
const itemList = [
"Apple",
"Orange",
"Banana",
"Cherry",
"Milk",
"Peanuts",
"Butter",
"Tomato"
];
const [filteredList, setFilteredList] = new useState(itemList);
const filterBySearch = (event) => {
// Access input value
const query = event.target.value;
// Create copy of item list
var updatedList = [...itemList];
// Include all elements which includes the search query
updatedList = updatedList.filter((item) => {
return item.toLowerCase().indexOf(query.toLowerCase()) !== -1;
});
// Trigger render with updated values
setFilteredList(updatedList);
};
return (
<div className="App">
<div className="search-header">
<div className="search-text">Search:</div>
<input id="search-box" onChange={filterBySearch} />
</div>
<div id="item-list">
<ol>
{filteredList.map((item, index) => (
<li key={index}>{item}</li>
))}
</ol>
</div>
</div>
);
}
styles.css
.App {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
font-size: 22px;
}
.search-header {
display: flex;
}
.search-text {
margin-right: 10px;
font-size: 22px;
display: flex;
align-items: center;
justify-content: center;
}
#search-box {
height: 25px;
}
#search-box[placeholder] {
line-height: 25px;
font-size: 25px;
}
#item-list {
min-height: 260px;
}