React is one of the top choices for JavaScript framework along with Angular however unlike Angular, React is considered more beginner-friendly with a small learning curve. As a beginner, learning React from scratch can be challenging, due to the sheer amount of concepts to cover.
A roadmap for learning React provides an overview of all main concepts of React along with the order in which it should be covered. A beginner who is starting or in progress with React can use the roadmap to make sure that the topics are evenly covered.
The prerequisites to learn React JS is:
- Knowledge of HTML to create a webpage using JSX code.
- Intermediate level of CSS to design React pages.
- Strong knowledge in core JavaScript, as React is a JavaScript library and requires code to be written in JavaScript.
- Basic knowledge in browser functionalities like loading speed, local storage, etc.
1. Learning React Virtual DOM
React framework is primarily used for creating a dynamic webpage, we may need some context to understand why? During JavaScript DOM manipulation, any change in an element of the page results in updating the whole DOM. The reason for DOM being so inefficient is it was designed for older 10-15 years old websites where updating DOM was less frequent as a result didn’t impact the overall performance.
The virtual DOM is a “Virtual” representation of real JavaScript DOM as JS object in React JS. React maintains a copy of HTML DOM in object format and performs the update on the copy rather than the original copy. Updating virtual copy doesn’t involve calling actual DOM methods thus saving time and improving performance. Finally, React compares real DOM with virtual DOM then updates only those objects where changes are required. (Refer codecademy.com/articles/react-virtual-dom for more info on virtual DOM)
In a static webpage, there isn’t much DOM manipulation, so using vanilla JavaScript will not make any difference. However, in a Dynamic webpage where DOM is manipulated frequently, vanilla JavaScript will significantly impact the page performance. Thus building dynamic webpages using React eliminates unnecessary updates due to the implementation of virtual DOM.
2. Understanding why and when to choose React JS
One of the most common the topic everybody misses out on is the main purpose of React JS and the type of projects where React must be adopted. As mentioned above first instance where React is particularly effective when used for developing dynamic webpages.
The second instance is when developing a single-page application is most ideal. Most of the JavaScript-based frameworks including React are used to develop single-page applications. A single-page application is a type of web app where the content is dynamically rewritten instead of reloading the whole page in contrast to a traditional webpage. (Refer easternpeak.com/why-choose-reactjs/ for more info)
Q: Best ways to learn react ?
- Learning Javascript first make understanding React easier
- Join online/offline React JS training bootcamp classes
- Free/paid React JS video crash from Udemy, YouTube, etc.
- React JS based ebooks, online tutorials and articles for reference.
- Building simple JavaScript based applications on React JS like calculator, Todo list, etc.
- Completing React coding challenges and certification tests.
3. Reuse Code by using React Components
In React a webpage is divided into several components, these components can be reused on multiple pages. For instance, A component for the navbar can be reused for the whole website thus saving development effort and also eliminate reloading duplicate code. Learn more about React components from medium.com/understanding-react-components.
To demonstrate the use of React Components let’s consider a list of static webpages with 3 sections for the top navbar, sidebar, and main body section of the page. Thus in React implementation, we divide the page into three components:
- Navbar: Display navigation menu consisting of all pages and when user clicks on an item, direct to respective page URL.
- Footer: Display the content of page footer like copyright, support email, etc which is constant across all web pages.
- PageBody: Render the body of every page based on the current active URL.
import React from "react";
import { Link } from "react-router-dom";
const NavBar = () => (
<div class="navbar-container">
<Link to="/page1">Menu 1</h1>
<Link to="/page2">Menu 2</h1>
<Link to="/page3">Menu 3</h1>
</div>
);
export default NavBar;
In App.js of the React project, we import all the React components and include them in the JSX code which is returned by the main App component.
import React from "react";
import { BrowserRouter as Router } from "react-router-dom";
// Import components
import NavBar from "./NavBar";
import Sidebar from "./Sidebar";
import PageBody from "./PageBody";
const App = () => (
<Router>
<NavBar/>
<Sidebar/>
<PageBody/>
</Router>
);
export default App;
React Component has the following advantages over static webpages:
- Reuse the code by dividing the page into components that can be reused on multiple pages.
- React only updates the component which is modified on user interactions such as navigating to a different page, etc.
- React components are loaded once and reused for multiple subsequent pages without reloading.
4. React lifecycle methods
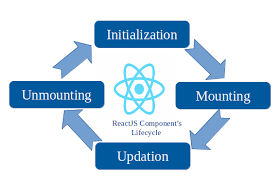
Whenever a page is loaded in React it executes lifecycle methods in a specific order. The lifecycle methods are divided into 3 main phases: Mounting, unmounting, and updating. Mounting life cycle methods such as componentDidMount(), getDerivedStateFromProps(), etc are triggered before initial render() call ie when the component is being mounted.
Updating life cycle methods such as shouldComponentUpdate(),componentDidUpdate(), etc are triggered on `setState()` calls which involves rendering the page to update values. Unmounting life cycle consists of componentWillUnmount() which will trigger at the end of page lifecycle.
For example, we are creating a web app that updates the page only on a specific condition then the condition should be validated in shouldComponentUpdate() to return True or False for updating the page. Learn more from programmingwithmosh.com/react-lifecycle-methods/.
5. React State and Props
Due to a webpage in React being a composition of multiple components, there needs to be a way to communicate among them. Here `Props` comes into play, Which is a feature that allows parent components to pass objects to child components.
A page has to be rendered for values to be updated. In case we use a standard JavaScript variable, any update on JavaScript variable value will not trigger, hence new values will not reflect on the webpage. React state will be used to store such variables together and setState() is used to update its values. Unlike JavaScript variables, setState() will trigger render()
thus reflecting the change on the webpage. Learn more about React state and props from freecodecamp.org/react-js-for-beginners-props-state-explained/
React Props | React State |
---|---|
1. React props are immutable, ie props cannot be updated within the child component. | 1. React states are mutable, ie can we updated using the setState() method. |
2. Props are passed from parent component to child component. | 2. States are declared for internal use within the component, however React state can be passed as props. |
3. Props cannot be a React state in React component. | 3. State can be assigned as Props for the child component. |
6. React Hooks and useEffect
From React 16.8 onward function-based component was introduced which works similar to class-based components but with some syntactical differences. In function `this` is undefined, hence object and methods are called directly, additionally this.state() and this.setState() was replaced with `useState()`.
The whole class-based React lifecycle is replaced with a single useEffect() method. Read more on React Hooks and useEffect() from flaviocopes.com/react-hook-useeffect, freecodecamp.org/react-components-jsx-props-for-beginners/.
The three features of useEffect() are as follows:
- The callback function of useEffect() is called every time the value of any of the dependencies is changed.
- Updating a state/variable included in useEffect() dependency array through the callback function will lead to an infinite loop.
- The callback function of useEffect() is executed at least once when the components are loaded.
- The useEffect() can only be declared in the React Hooks function.
7. React useContext
When there multiple levels of child component receive props from topmost parent to bottom-most child component can be very cumbersome. Hence in such cases useContext() comes into play, where you need to specify the context objects once from the main parent component and it will accessible from any child component irrespective of their levels. Learn more about useContext() from daveceddia.com/usecontext-hook/ .
Here are the following cases where useContext() can be used:
- When React State, variable, etc needs to be accessed from multiple levels of nested React components.
- Avoid Props to be passed through multiple levels of child components.
Conclusion
Finally, we can conclude that the React Roadmap lays down all the topics that are most important for anyone who is starting or in progress to learn React.