In this article, we will learn how to use Array.reduce() on Array of objects and types of functionalities that can be accomplished using Reduce() in JavaScript.
So you might ask when do we need to use Reduce()? The answer is quite simple, we much use Array.reduce() to generate a single value array of values unlike other JavaScript array methods like map(), filter(), etc which return transformed array as result.
First, let’s discuss the syntax for using reduce() on an array of objects to access each item and perform functionalities.
array.reduce(
// Callback Function
(reduceResult, item, index) => {
//Access item object
//Return reduce result for next index
},
//Initial reduceResult Value
initReduceResult
);
In the above syntax, we access reduceResult, item, and index from the function parameter in the callback function. Inside the callback function, we will return reduceResult value for the next iteration through the callback function. Further, we can pass the initial value for “initReduceResult” as the second parameter in Array.reduce().
Let’s consider an example of a list of books, the book itself has many properties such as the name of the book, no of pages, date of publication, etc. In this article, we will demonstrate the following functionalities:
- Calculating total of number pages in the whole list by adding page number from each book.
- Finding lowest and higest page number count from the list.
- Finding book with lowest and higest page number in the list.
- Finding oldest book in the list.
- Finding latest date of publication value from the list.
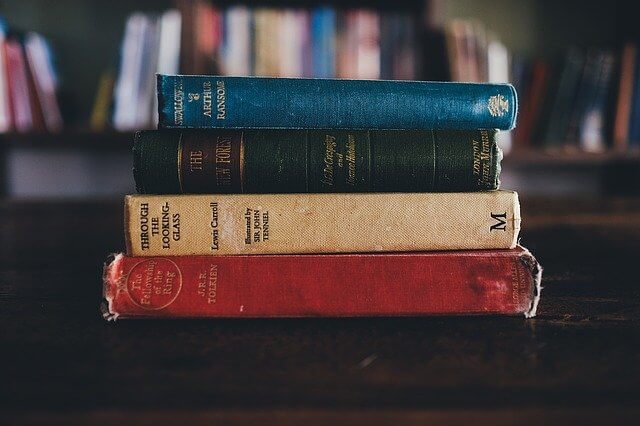
For the code-based representation of the list of books, we are considering the array of books with property names “name”, “no_pages” and “release_date”. Also, the release_date property is in “YYYY-MM-DD” format to allow the JS date object to parse the string.
const bookList = [{name: "Harry Potter", no_pages: 700, release_date: "2010-11-20"},
{name: "Da Vinci Code", no_pages: 800, release_date: "1990-03-04"},
{name: "Breaking Dawn", no_pages: 600, release_date: "2001-08-14"},
{name: "Eclipse", no_pages: 1200, release_date: "2006-05-22"}]
Calculating the Sum of a property using reduce()
To calculate the sum, we need to return total + item.propertyValue from the callback function. Additionally, it is important to initialize the total to 0 by passing 0 as the second parameter of reduce. The default value of total is undefined, hence total+ item.propertyValue will throw an error if the initial value is not provided.
In the below example, we are calculating the total of “no_pages” property from each book object present in bookList array. Returning “item.no_pages + total” ensures each “no_pages” property is added at every iteration.
// Calculate total sum of no_pages property
const totalPages = bookList.reduce((total, item) => item.no_pages + total, 0);
console.log(totalPages) //3300
Finding Minimum and Maximum property value using reduce()
We can find maximum or minimum property values from the array by returning min or max values at each instance. The initial value is set to array[0].property_name as adding “0” or using any other value might result in the initial value being the minium thus providing wrong results.
In the below example, we are finding the min and max page number from a book by returning “return min > item.no_pages ? item.no_pages: min” for the minimum and “return min < item.no_pages ? item.no_pages: min” for the maximum respectively.
// Finding min "no_pages" value from the array
const minNoOfPages = bookList.reduce(
(min, item) => {
return min > item.no_pages ? item.no_pages: min
}, bookList[0].no_pages);
console.log(minNoOfPages) //600
// Finding max "no_pages" value from the array
const maxNoOfPages = bookList.reduce(
(min, item) => {
return min < item.no_pages ? item.no_pages: min
}, bookList[0].no_pages);
console.log(maxNoOfPages) //1200
Finding Object with Min and Max property value using reduce()
In some cases where we need to access other property values of the min/max object rather than the min/max value itself, finding the whole object would be more suitable. We accomplish that by returning the “item” itself in contrary to “item.property_value”.
In the below example, we are finding the object with min and max no_pages values from bookList.
// Finding object with minimum no_pages value
const bookMinPage = bookList.reduce(
(min, item) => {
return min.no_pages > item.no_pages ? item : min
}, bookList[0]);
console.log(bookMinPage)
//{name: "Breaking Dawn", no_pages: 600, release_date: "2001-08-14"}
// Finding object with minimum no_pages value
const bookMaxPage = bookList.reduce(
(min, item) => {
return min.no_pages < item.no_pages ? item : min
}, bookList[0]);
console.log(bookMaxPage)
//{name: "Eclipse", no_pages: 1200, release_date: "2006-05-22"}
Finding Min and Max Date values from the array
In order to find the minimum and maximum Date values among an array of objects, we need to first parse the string value using the new Date() or Date.parse() before comparing it with the JavaScript operator.
In the below example, we are finding the book with the newest release date and the oldest release date value among books in bookList. Read more about JavaScript Date object from developer.mozilla.org/JavaScript/Global_Objects/Date
// Finding the object with latest "release_date" property value
const latestBook = bookList.reduce(
(min, item) => {
const currentLatest = new Date(min.release_date);
const itemLatest = new Date(item.release_date);
return currentLatest > itemLatest ? min : item;
}, bookList[0]);
console.log(latestBook);
//"{name: "Harry Potter", no_pages: 700, release_date: "2010-11-20"}"
// Finding the lowest date value in "release_date" property
const oldestBookDate = bookList.reduce(
(min, item) => {
const currentLatest = new Date(min);
const itemLatest = new Date(item.release_date);
return currentLatest > itemLatest ? item.release_date : min;
}, bookList[0].release_date);
console.log(oldestBookDate);
// "1990-03-04"