In this article, you will learn how to display every item from the list/array on-screen in React JS. The array is rendered by the following steps:
- Apply Array.map() on the variable with array reference.
- Access the every item and index through callback function.
- Return JSX every item with index as the key.
To demonstrate the above steps we will consider an example to render an array of string values in React JS. The output for this example is shown below where every string value of the list is printed in ascending order.
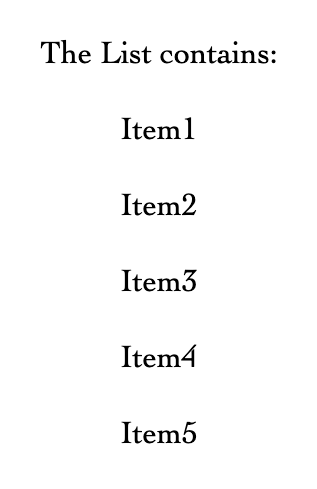
1. Declare JS constant with List of items
The first step is to declare JS constant assigned with an array of strings. In the code below, we will declare five-string values ranging from “Item1” to “Item5”.
const itemList = ["Item1", "Item2", "Item3", "Item4", "Item5"];
2. Generate JSX code for each item
To generate JSX code that renders the array, Array.map() function is applied on previously declared itemList constant with a callback function. In the callback function, the string value of the array entry is accessed, and <div></div> enclosing item string value is returned.
// Generate JSX code for Display each item
const renderList = itemList.map((item) =>
<div>{item}</div>
);
3. Add key for every item in the list
React JS requires a unique key for every dynamic array JSX element, this allows React virtual DOM to identify changes in the array such as update, delete, etc more efficiently.
If the key is not added then you will see a console error with the message as “Each child in an array or iterator should have a unique “key” prop“.
Learn more about List and Keys in React JS from reactjs.org/docs/lists-and-keys.html.
// Add array index as the key
const renderList = itemList.map((item, index) =>
<div key={index}>{item}</div>
);
4. Include JSX code in the App component
The final step is to include the previously declared constant renderList in the JSX code returned by the component.
return (
<div className="app">
<div>The List contains:</div>
{renderList}
</div>
);
Final Solution Code
App.js
import React from "react";
import ReactDOM from "react-dom";
import "./styles.css";
function App() {
const itemList = ["Item1", "Item2", "Item3", "Item4", "Item5"];
// Generate JSX code for Display each item
const renderList = itemList.map((item, index) =>
<div key={index}>{item}</div>
);
return (
<div className="app">
<div>The List contains:</div>
{renderList}
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
styles.js
.app {
font-family: sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
flex-direction: column;
gap: 20px;
height: 100vh;
font-family: Cambria, Cochin, Georgia, Times, "Times New Roman", serif;
}