In this article, we will demonstrate looping through an array of objects in JavaScript with a practical example of an employee list. In the below example, each employee has properties of name, position, profile_url, and tags. Since we are considering a list of employees, all the employee objects will be enclosed in a JS array.
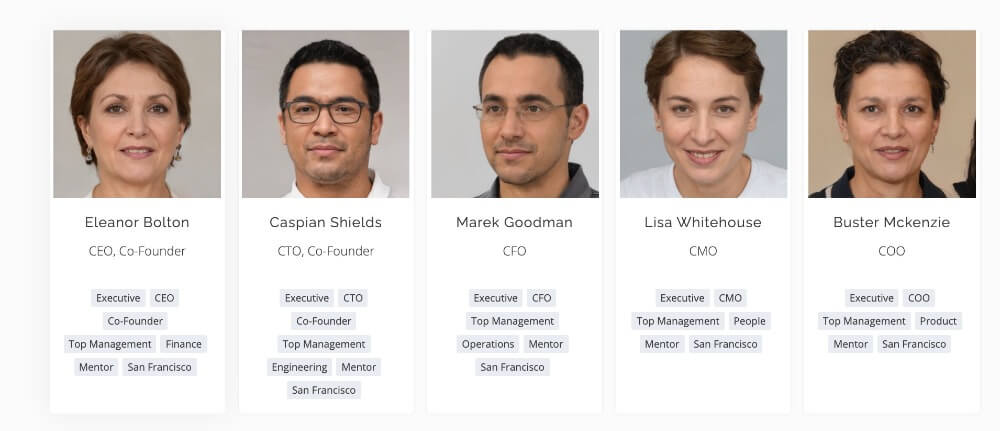
The JavaScript-based representation as an array of objects of the above example is as follows:
let employeeList = [
{first_name:"Eleanor", last_name:"Bolton", designation:"CEO, Co-Founder", tags: ["Finance","San Francisco", "Mentor", "Top Management"], age:45},
{first_name:"Caspian", last_name:"Shields", designation:"CTO, Co-Founder", tags:["Engineering","San Francisco", "Mentor", "Top Management"], age:34},
{first_name:"Marek", last_name:"Goodman", designation:"CFO", tags:["Operations","New York", "Mentor", "Top Management"], age:31},
{first_name:"Lisa", last_name:"Whitehouse", designation:"CMO", tags:["People","San Francisco", "Mentor", "Top Management"], age:39},
{first_name:"Buster", last_name:"Mackenzi", designation:"COO", tags:["Product","New York", "Mentor", "Top Management"], age:43}
];
How to loop through an Array of Objects
- Array.forEach() for simple iteration.
- Array.map() to transform every object of the array.
- Array.filter() to create subset of objects from the array.
- Array.reduce() to combine array elements into single object.
- Array.every() to all object in the array satisfy a condition
- Array.sort() to reorder the list of objects
- Array.find() to search for an object in the array
The above are different ways to iterate over an array of objects, we will discuss each individual method in detail later in this article. Iterating over an array of objects itself is very similar to a simple array iteration, however, operation on array objects is more complex as each item is an object as compared to a string or number.
Simple iteration over array of objects
Accessing items of an array of objects is accomplished using the Array.forEach() method with a callback function where every object in the array can be accessed as a parameter. However, unlike simple array iteration, the parameters are objects hence its properties can be destructured directly if required.
In the below example, we access each employee object from employeeList and destructure “first_name”, “last_name”, and “designation” properties. Additionally, we will print details on the screen using a console log statement.
// Print first name, last name and designation of every employee on the list
employeeList.forEach(({first_name, last_name, designation})=>{
console.log(`${first_name} ${last_name} is the ${designation}`)});
// "Eleanor Bolton is the CEO, Co-Founder"
// "Caspian Shields is the CTO, Co-Founder"
// "Marek Goodman is the CFO"
// "Lisa Whitehouse is the CMO"
// "Buster Mackenzi is the COO"
Transform Array of Objects into a new array
An array of objects can be transformed using the Array.map() method which is a higher-order function, refer dev.to/higher-order-functions to learn more about higher-order function. It is important to note that Array.map() doesn’t modify the original array and instead returns the transformed array.
In the below example, we create a new array that consists of all the employee names by using Array.map() on employeeList object. In the callback function, we return the combined value of both first_name and last_name properties. The final result will be an array of string values of employee names from employeeList.
// Create new array from employeeList with all employee names
const employeeNames = employeeList.map(
({first_name, last_name})=> first_name + " " + last_name);
console.log(employeeNames);
// ["Eleanor Bolton", "Caspian Shields", "Marek Goodman", "Lisa Whitehouse", "Buster Mackenzi"]
Add or delete property in array of objects
To add a new property to every object of the array, we need to use array.map() similar to transforming array but the value is assigned to the same array instead of a new array. Further, we need to use the spread operator to retain existing properties which should be followed by new property key/value pairs that need to be added, ie {…object, newProperty: propertyValue}. Read more about spread operator from developer.mozilla.org/Spread_syntax.
In the below example, we are adding the “name” property to every object in employeeList array with value as string concatenation of first_name and last_name properties. The value of employeeList array is updated with the value returned by the employeeList.map() function.
// Add "name" property to all the objects in the list
employeeList = employeeList.map((employee)=> {
return {...employee, name: employee.first_name + " " + employee.last_name}});
console.log(employeeList);
In the example below, the deletion of property “tags” from every object in employeeList array is achieved by the Array.forEach() method. In the callback function, we access every object as a parameter, and “delete employee.tags” ensures that the tags property is deleted from every object reference in employeeList array. Read more about the delete operation from developer.mozilla.org/Operators/delete.
// Delete "tags" property from all the objects in employeeList
employeeList.forEach((employee)=> {
delete employee.tags
});
console.log(employeeList);
Sum of a property in array of objects
The sum of the properties of objects in the array is calculated by using Array.reduce() method. The main function of Array.reduce() is to derive a single value from the whole array, in this case, to generate the total sum from all array entries.
In the below example, we apply to reduce on employeeList with initial value as an object with property age with 0 value. In the callback function, we add “total.age” with the value of the “age” property from object entry.
// Calculate total age to find the average age of employee list
const empTotal = employeeList.reduce(
(total, {age})=> {
total.age = total.age + age;
return total;
}, {age:0})
console.log(empTotal) //{age: 192}
const avgAge = empTotal.age/employeeList.length;
console.log(`Average age of employees are: ${avgAge}`);
// "Average age of employees are: 38.4"
Search an element in array of objects
We can accomplish search functionality in an array of objects using Array.find() and match every object with search object values. The Array.find() method returns the first matched object from the object array.
In the below example, the “first_name” property of objects from employeeList is matched with “Caspian” to find an employee with the first name as “Caspian”. The first object that returns true for `employee.first_name == “Caspian”` condition is returned and assigned to searchedEmployee JavaScript constant.
// Search employee with first name as "Caspian"
const searchedEmployee = employeeList.find(
(employee) => employee.first_name == "Caspian");
console.log(searchedEmployee);
// {first_name:"Caspian", last_name:"Shields", designation:"CTO, Co-Founder",
// tags:["Engineering", "San Francisco", "Mentor", "Top Management"], age:34}
Filter elements based on a criteria
The array items can be filtered using Array.filter(), however in contrast to a simple array, the elements are matched using the property value of every object.
In the below example, We need to find all the employees who are from “New York” through tags property in the object entry. The employeeList array is filtered using the condition whether the “New York” string is present in the tags property array of the object.
// Find subset of employees who are from New York
const employeesInNewYork = employeeList.filter(
(employee)=> employee.tags.indexOf("New York") != -1);
console.log(employeesInNewYork);
// [{first_name:"Marek", last_name:"Goodman", designation:"CFO",
// tags:["Operations","New York", "Mentor", "Top Management"], age:31},
// {first_name:"Lisa", last_name:"Whitehouse", designation:"CMO",
// tags:["People","San Francisco", "Mentor", "Top Management"], age:39},
// {first_name:"Buster", last_name:"Mackenzi", designation:"COO",
// tags:["Product","New York", "Mentor", "Top Management"], age:43}]
Sort elements in array of objects
The Array.sort() method allows an array to be sorted based on the criteria provided in the callback function. Unlike a simple array, an array of objects can be sorted based on any single or multiple properties of the object entry.
In the below example, we sort employeeList by ascending order of the “age” object property. The condition “emp1.age – emp2.age” returns a negative value when two objects of “age” property values are not sorted and thus rearranging the array.
// Sort employees by ascending order of age
employeeList.sort((emp1, emp2)=> emp1.age - emp2.age);
console.log(employeeList);
Validate every object entries
The Array.every() accomplishes validation of an array and returns a boolean value based on whether the condition is satisfied by all the objects of the array.
In the below example, we check if every employee is above the age of 18 years or not. If employeeList.every() method will return true only if every object satisfies “employee.age > 18” condition.
// Check if every employee are over 18 years
const areEmployeesOver18 = employeeList.every((employee)=> employee.age > 18);
if(areEmployeesOver18){
console.log("All the employees are over 18 years");
}
else {
console.log("1 or more employees are underaged");
}