In this article, we will learn how to build an Increment Counter app using React JS hooks, which has the following features:
- Display initial count as 0 on page load
- Display Button to increment the count
- Increment count by 1 on button click
- Display the updated count on the screen
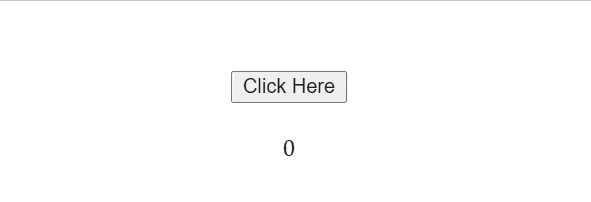
1. Declare React State to store the count value
First, we need to declare a React State to store the value of the count. The actual value is accessed through “count“ state and setCount() method updates the “count” state with the value passed as the parameter.
// State to store count value
const [count, setCount] = useState(0);
2. Display count on screen
The count state is rendered on the screen by including it into the JSX code within the ‘{ }’ braces. The JSX code returned by the component is parsed into the HTML code and included in the webpage.
return (
<div className="app">
{count}
</div>
);
3. JS Function to increment count
We will declare a JS function to increment the count by calling setCount() method. We pass “count + 1” value to setCount() method as parameter, which updates “count” state value. The setCount() method archives two tasks assign incremented value to the “count” React state and render updated value on screen.
// Function to increment count by 1
const incrementCount = () => {
// Update state with incremented value
setCount(count + 1);
};
4. Add Button with onClick to trigger increment
Finally, we need to integrate all the code together to allows users to trigger the increment count functionality. This is achieved by creating an HTML button with the previously implemented incrementCount function as the onClick attribute.
return (
<div className="app">
<button onClick={incrementCount}>Click Here</button>
{count}
</div>
);
Final Solution Code
App.js
import React, { useState } from "react";
import ReactDOM from "react-dom";
import "./styles.css";
function App() {
// State to store count value
const [count, setCount] = useState(0);
// Function to increment count by 1
const incrementCount = () => {
// Update state with incremented value
setCount(count + 1);
};
return (
<div className="app">
<button onClick={incrementCount}>Click Here</button>
{count}
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
styles.css
.app {
font-family: sans-serif;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
gap: 20px;
height: 100vh;
font-family: Cambria, Cochin, Georgia, Times, "Times New Roman", serif;
}