In this article, we will learn how to use session storage in React JS with a page view counter app. The session storage has the following features:
- Persists data for only current active tab of the application.
- Data is reset for new tab in same window.
- Data is cleared when current window is closed.
We read and write data to sessionStorage through the steps below:
// Access value associated with the key
var item_value = sessionStorage.getItem("item_key");
// Assign value to a key
sessionStorage.setItem("item_key", item_value);
Note: All values in Session storage will be stored in string format, hence must be parsed to other data types if required.
We will consider the example of building the page view counter app that keeps track of each pageview by storing the value in sessionStorage and incrementing the view count in page load. The React code is placed in UseEffect() in order to trigger it at each page load.
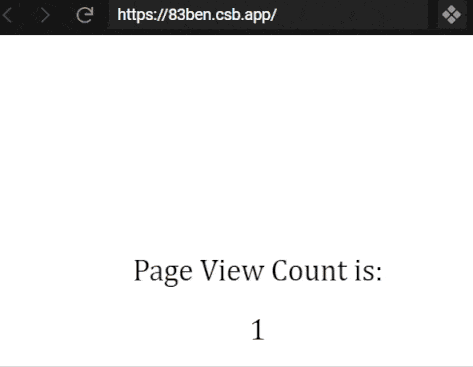
1. Declare React state for page view count
We will declare React state to track page view count with 0 as the initial value. Updating the count using setCount() ensures that the values are updated dynamically on screen.
const [count, setCount] = useState(0);
2. Display the view count on screen
The count is displayed on the screen by including {count} in JSX code which is returned by App component.
return (
<div className="app">
<div>Page View Count is:</div>
{count}
</div>
);
3. Initialize view count in session storage
Next, we will add useEffect() with no dependencies to trigger React code on page load. The page view count is initialized to 1 on page load. Learn more about React useEffect() from dmitripavlutin.com/react-useeffect-explanation/
useEffect(() => {
// Initialize page views count
var pageView = 1;
// Update the state
setCount(pageView);
}, []);
4. Access view count from session storage
In order for the page view counter to work, we need to introduce session storage to persist values beyond page reload.
- The existing value is accessed from sesstion storage with key “pageView”.
- If entry doesn’t exist ie pageView = null, initialize pageView variable to 1.
- else increment the pageView value by 1.
- Finally, update the session storage value for next page reload.
useEffect(() => {
// Access initial value from session storage
var pageView = sessionStorage.getItem("pageView");
if (pageView == null) {
// Initialize page views count
pageView = 1;
} else {
// Increment count
pageView = Number(pageView) + 1;
}
// Update session storage
sessionStorage.setItem("pageView", pageView);
setCount(pageView);
}, []); //No dependency to trigger in each page load
Final Solution Code
App.js
import React, { useEffect, useState } from "react";
import ReactDOM from "react-dom";
import "./styles.css";
function App() {
// React State
const [count, setCount] = useState(0);
useEffect(() => {
// Access count value from session storage
var pageView = sessionStorage.getItem("pageView");
if (pageView == null) {
// Initialize page views count
pageView = 1;
} else {
// Increment count
pageView = Number(pageView) + 1;
}
// Update session storage
sessionStorage.setItem("pageView", pageView);
setCount(pageView);
}, []); //No dependency to trigger in each page load
return (
<div className="app">
<div>Page View Count is:</div>
{count}
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
styles.css
.app {
font-family: sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
flex-direction: column;
gap: 20px;
height: 100vh;
font-family: Cambria, Cochin, Georgia, Times, "Times New Roman", serif;
font-size: 25px;
}