In this article, we will learn how to use a javascript Array.map() function on an array of objects to perform different types of functionalities like accessing, transforming, deleting, etc. List of functions you can perform with the Array.map() function are:
- Simple iteration over array of objects.
- Create new trasformed array.
- Get list of values of a object property.
- Add properties to every object entry of array.
- Delete a property of every object entry in array.
- Access key/value pairs of each object enty.
We will consider an example of a to-do list which can be represented as an array of each item object with properties of the item name, price, quantity, isChecked, and brand details.
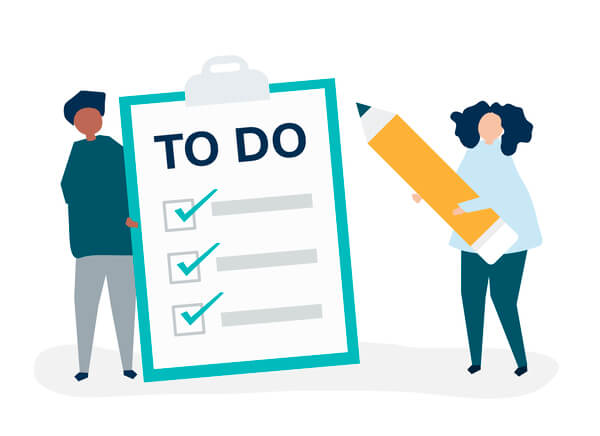
The array of objects representation of todo list is JavaScript is as follows:
let todoList = [
{item_name:"Apple", price:"$5", quantity:1, brand_details: {name:"Golden Delicious", location:"San Francisco"}, isChecked:true},
{item_name:"Bannana", price:"$3", quantity:2, brand_details: {name:"Fuji", location:"San Diego"}, isChecked:false},
{item_name:"Pears", price:"$7", quantity:4, brand_details: {name:"Golden Delicious", location:"San Francisco"}, isChecked:true},
{item_name:"Milk", price:"$4", quantity:3, brand_details: {name:"Mother Dairy", location:"California"}, isChecked:false},
];
Simple Iteration of an Array of Objects
Every object from an array of objects is accessed as a function parameter in the callback function of Array.map(), the object properties can be de-structured or access directly as the item.property_name. The objects can also be accessed using Array.forEach(), however unlike Array.map() it always returns undefined instead of a new transformed array.
In the below example, item_name, price, and quantity object properties are accessed and logged for every object in an array of objects using Array.map(). The second example is similar but the only item_name with the brand name of objects satisfying isChecked=true condition are logged on the screen.
// Print item_name, price and quantity of each object in todo list
todoList.map(({item_name, price, quantity})=>{
console.log(`${item_name} with quantity ${quantity} with price ${price}`)
});
// "Apple with quantity 1 with price $5"
// "Bannana with quantity 2 with price $3"
// "Pears with quantity 4 with price $7"
// "Milk with quantity 3 with price $4"
// Print item_name and brand name of all checked object
todoList.map(({item_name, isChecked, brand_details: {name}})=>{
if(isChecked){
console.log(`${item_name} from ${name} is selected`);
}
});
// "Apple from Golden Delicious is selected"
// "Pears from Golden Delicious is selected"
Transforming Array of Objects using map()
The main function of Array.map() is to transform an array into a new array with different values. The entries for the transformed array are returned from the callback function of Array.map().
In the below example, a 2D array is constructed from todoList array by returning an array with item_name and isChecked values in the Array.map() callback function. Similarly in the second example, we can construct an array with object entires of item_name and price from todoList declaration.
// Transform into nested array of item_name with isChecked value
todoList.map(({item_name, isChecked})=>{
return [item_name, isChecked];
});
// [["Apple", true]
// ["Bannana", false]
// ["Pears", true]
// ["Milk", false]]
// Transform into array of objects with item_name and price
todoList.map(({item_name, price})=>{
return {item_name, price};
});
// [{item_name: "Apple", price: "$5"}
// {item_name: "Bannana", price: "$3"}
// {item_name: "Pears", price: "$7"}
// {item_name: "Milk", price: "$4"}]
Creating List from Array of Objects
A list of all property values from the array of objects can be retrieved using Array.map() by returning the property value in the callback function. In the below example we create an array of all item_name values using Array.map() on todoList declaration. The second example demonstrates creating an array of brand location values which is very similar to the first with another level of de-structuring for accessing brand location value.
// Print all item_name values from array of objects
const itemList = todoList.map(({item_name})=> item_name);
console.log(itemList)
// ["Apple", "Bannana", "Pears", "Milk"]
// Print unique brand location values from array of objects
let productLocations = todoList.map(({brand_details: {location}}) => location);
productLocations = new Set(productLocations)
console.log(productLocations)
// {"San Francisco", "San Diego", "California"}
Add Property to Array of Object
In order to add a new property to every object from the array of objects, the transformed object with a new property value has to be returned in the Array.map() callback function. We use the JavaScript spread operator to include all existing object property values along with the new properties that need to be added to object entries. Read more about spread operator from developer.mozilla.org/Operators/Spread_syntax.
In the below example, we are adding “total” property with values as total = price*quantity for every object entry of todoList array. Learn more about the Number() method from developer.mozilla.org/JavaScript//Number
// Adding "total" property to todoList objects
todoList = todoList.map(
(item)=> {
// Parsing price string to number value
const price = Number(item.price.replace("$", ""))
return {...item, total: `\$${price*item.quantity}`}
});
console.log(todoList)
Delete Property from Array of Object
There are two approaches to delete a property from an array of objects using Array.map(), they are as follows:
- Update array with new transformed array by returing an object without the property in the callaback function inside Array.map().
- Iterate over every object and perform “delete object.property_name” to permanently delete the property from the reference object.
In the below example, the “brand_details” property is deleted from todoList array by excluding it in the return object of the callback function inside Array.map(). Further, we delete the “price” property by performing “delete item.price” on every object reference of todoList array.
// Transform array to exclude brand_details property from todoList
todoList = todoList.map(
({item_name, price, quantity, isChecked})=> {
return { item_name, price, quantity, isChecked }
});
console.log(todoList)
//[{item_name: "Apple", price: "$5", quantity: 1}
// {item_name: "Bannana", price: "$3", quantity: 2}
// {item_name: "Pears", price: "$7", quantity: 4}
// {item_name: "Milk", price: "$4", quantity: 3}]
// Delete price property for every object entry in todoList
todoList = todoList.map(
(item)=> {
delete item.price
return item;
});
console.log(todoList)
// [{item_name: "Apple", quantity: 1}
// {item_name: "Bannana", quantity: 2}
// {item_name: "Pears", quantity: 4}
// {item_name: "Milk", quantity: 3}]"
Accessing each Key/Value Pair in Array of Objects
The key/value pairs of object entries can be accessed using Object.entries() method alternatively, the list of keys/values can be accessed individually using Object.keys() and Object.values(). Learn more about Object.entries() from developer.mozilla.org/Object/entries
In the below example, we will create an array of key/value pairs of object entries in todoList array using Object.entries() with Array.map(). Further, we used nested Array.map() to convert brand_details object into key/value pair array.
// Getting key/value pair of object entries in todoList array
todoList.map((item)=>{
return Object.entries(item).map(
(todoList_entry)=>{
// Getting key/value pair of brand_details object
if(todoList_entry[0]=="brand_details"){
return Object.entries(todoList_entry[1]).map(
(brand_details_entry)=> brand_details_entry);
}
else{
return todoList_entry;
}
})
});
// [["item_name", "Apple"], ["price", "$5"],
// ["quantity", 1],
// [["name", "Golden Delicious"],
// ["location", "San Francisco"]],
// ["isChecked", true]]
...(more)