In JavaScript, an object is represented as the list of entries with key-value pairs. Removing a key-value pair from the list results in the object’s property begin excluded. Deleting a property directly will result in permanent loss of key-value pair and cannot be restored or accessed in the future. In scenarios where the deleted values need to be accessed, you have to maintain an original copy of the JavaScript object.
var person = {
name: "John Doe",
prefix: "Mr",
age: 30
}
The person JavaScript object contains two properties of “name” and “age” described as key-value pairs. Once you have deleted the “age” property, the value of “person.age” will be undefined.
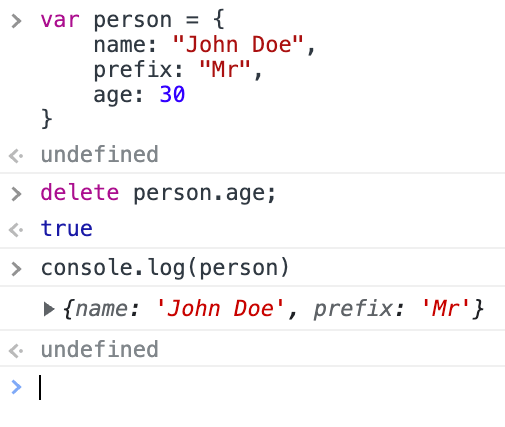
1. Delete or remove property permanently
The “delete” keyword permanently removes a property from the JavaScript object. Once the delete operation is applied, the object behaves as if the property is never defined in the first place.
// Syntax
delete object.property_name
In the below example, the “age” property of the person object is removed using the “delete” keyword. After the delete operation, the value of “person.age” will be undefined and the key-value pair mapped to the “age” property is erased from the object.
delete person.age
console.log(person.age)
// undefined
console.log(person)
// {name: 'John Doe', prefix: 'Mr'}
2. Destructure the object to exclude properties
The object destructuring extracts properties from an object and binds the values to the variables. When object destructuring is combined with the spread operator, the variable will be assigned to the object with non-destructured properties.
Destructuring allows a property to be excluded from the object without modifying the original object.
const {property_name, ...new_object} = original_object
The “updated_person” object contains all properties of the “person” object excluding the “age” property which is destructured before the “updated_person” object.
const {age, ...updated_person} = person
console.log(person)
// {name: 'John Doe', prefix: 'Mr', age: 30}
console.log(updated_person)
// {name: 'John Doe', prefix: 'Mr'}
3. Assign undefined to clear property values
In JavaScript, the “undefined” value equates to a property/variable not begin assigned a value. Hence, the value of “object.unkown_property” is always undefined.
Manually assigning “undefined” does not erase the key-value pair from the object which makes this approach less ideal. That said, JavaScript object to JSON conversion with the “JSON.stringify()” method ignores properties with the undefined value.
The “age” property of the person object is assigned with the undefined value, as a result, the key-value pair is not removed. “JSON.stringify(person)” converts the object into string format where the “age” property is ignored.
person.age = undefined
console.log(person.age)
// undefined
console.log(person)
// {name: 'John Doe', prefix: 'Mr', age: undefined}
console.log(JSON.stringify(person))
// {"name":"John Doe","prefix":"Mr"}
4. Reduce properties using Object.keys()
“Object.keys()” is an inbuilt JavaScript method that returns a list of keys associated with the object passed as the argument. “Array.reduce” is applied on the list return from “Object.keys()” method to combine the properties into a single object.
“Array.reduce” requires two parameters as follows:
- Callback function: Javascript function to add key-value pair to accumulator object and ignores properties that need to be removed.
- Initial object: Specify the initial value as an empty object for the accumulator object.
var updatedObject = Object.keys(person).reduce(
(acc, key)=>{
if(key != "age"){
acc[key] = person[key];
}
return acc;
},{});
console.log(updatedObject);
// {name: 'John Doe', prefix: 'Mr'}
5. Exclude properties using for…in loop
A simple approach to removing property is populating an empty object using for…in loop. We need to iterate over every key-value pair of the parent object and assign all required properties to a new object in addition to avoiding properties that need to be removed.
In the below example, we iterate over all keys of the person object using for…in loop. Inside the loop, the newObject variable is assigned with the property if key != ‘age’ is true, ensuring that the age property is not added to the object.
// Initialize empty object
var newObject = {};
for(key in person){
if(key != "age"){
newObject[key] = person[key];
}
}
console.log(newObject)
// {name: 'John Doe', prefix: 'Mr'}