1. Define Default Value
The fetch() call is an asynchronous method, hence we need to use a default value until the REST API returns a response. Defining incorrect default values will cause JavaScript errors even before the fetch() call is triggered, hence ensure that the default value is supported by the React JS code.
In our case, since we are expecting an array from an API call, the default value will be an empty array.
const defaultValue = []
2. Declare React state to store API data
First, we need to declare React State to store the list of users returned from the response of the API call. Updating the state through “setUsers()” ensures that screen is updated once list of users are received from the REST API call.
// Store list of all users
const [users, setUsers] = useState(defaultValue);
3. JS function to fetch API data and store the response
We need to declare a JS function to retrieve the data from an external URL in the following steps:
- We fetch the data from the “https://jsonplaceholder.typicode.com/todos/” URL using JavaScript fetch() API.
- Add a callback function to then() for converting the response into JSON format.
- The user React state is updated with the list of users from the API response.
// Function to collect data
const getApiData = async () => {
const response = await fetch(
"https://jsonplaceholder.typicode.com/todos/"
).then((response) => response.json());
// update the state
setUsers(response);
};
4. Add useEffect to fetch API on page load
Once we have defined the function to retrieve the API data, it needs to trigger on page load. The getApiData() added into React useEffect() with empty dependency array, which ensures code is triggered once on page load.
useEffect(() => {
getApiData();
}, []);
5. Generate JSX Code with React state
Finally, we need to display the details of every user. Hence we will generate JSX code for every user by using Array.map() and the callback function returns <div></div> with the user.id and user.title.
<div className="app">
{users.map((user) => (
<div className="item-container">
Id:{user.id} <div className="title">Title:{user.title}</div>
</div>
))}
</div>
6. API call through Axios
The main disadvantages of fetch() call is we have manually convert the data from API to JSON format. Hence, Axios NPM package is commonly used to automatically manage the JSON conversion. Learn more about fetch() vs axios from blog.logrocket.com/axios-vs-fetch-best-http-requests. Once “axios” is installed in package.json, the getAPIData() method can be implemented through axios as follows:
import axios from "axios";
// Function to collect data
const getApiData = async () => {
const response = await axios(
"https://jsonplaceholder.typicode.com/todos/"
);
// update the state
setUsers(response);
};
Final Solution Code
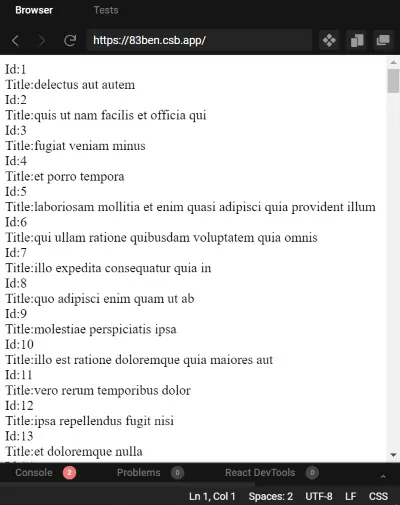
App.js
import React, { useEffect, useState } from "react";
import ReactDOM from "react-dom";
import "./styles.css";
function App() {
const defaultValue = []
const [users, setUsers] = useState(defaultValue);
// Function to collect data
const getApiData = async () => {
const response = await fetch(
"https://jsonplaceholder.typicode.com/todos/"
).then((response) => response.json());
setUsers(response);
};
useEffect(() => {
getApiData();
}, []);
return (
<div className="app">
{users.map((user) => (
<div className="item-container">
Id:{user.id} <div className="title">Title:{user.title}</div>
</div>
))}
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);