In this article, we will build a website visitor counter app with the following functionalities :
- Display the total number of visits on the page.
- Functionality to reset the number of visits on button click.
- Increment the count on refresh and future visits.
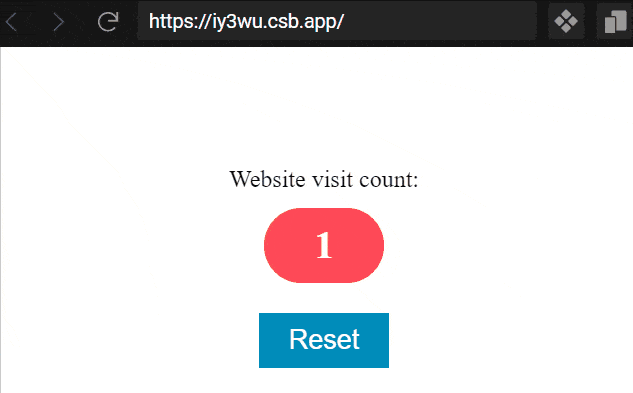
We will first start with creating HTML structure and then integrated it with JavaScript functionality which is followed by adding CSS styles.
1. Create <div> container to visitor count using HTML code
The title text is added to HTML file by adding wrapping it in <div></div>. In addition, we need to add another <div></div> with class “website-counter” with empty content for displaying visitor count using JavaScript.
<html>
<head>
<title>Website Counter</title>
<script defer src="index.js"></script>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div>Website visit count:</div>
<div class="website-counter"></div>
</body>
</html>
2. DOM querySelector() to match the container using JavaScript
The next step is to use document.querySelector() to match the HTML element with the class “website-counter” and assign it to a variable for future DOM manipulation operations. Learn more about querySelector from developer.mozilla.org/Document/querySelector.
var counterContainer = document.querySelector(".website-counter");
3. Retrieve visitor count using LocalStorage.getItem()
Now we retrieve the previous value of website visitor count from localStorage by using the localStorage.getItem() method by passing key “page_view” as a parameter. The value is stored in localStorage of the web browser and persists the data beyond a single session. Learn more about localStorage and its methods from tiny.cloud/blog/javascript-localstorage/.
var visitCount = localStorage.getItem("page_view");
4. Initialize visitor count to 1 in localStorage if entry is not found
The localStorage entry is absent during first user session, hence localStorage.getItem(“page_view”) will return undefined. This case is handled by initializing the visitCount to 1 and adding the entry using localStorage.setItem(“”) with “page_view” as the key.
visitCount = 1;
//Add entry for key="page_view"
localStorage.setItem("page_view", 1);
5. Increment visitor count and update the localStorage
On the contrary previous scenario, if an entry for the “page_view” key is already present, the retrieved String value is converted to a number datatype using Number(). The previous session visitCount is then incremented and the value is updated in localStorage.
visitCount = Number(visitCount) + 1;
// Update local storage value
localStorage.setItem("page_view", visitCount);
6. Display visitor count on page using element.innerHTML
Once the correct value of visitCount is determined, it needs to be rendered on the screen. This is accomplished by assigning the visitCount variable to element.innerHTML. The .innerHTML property on selected DOM element allows HTML code to be added inside selected DOM element. As a result, the HTML code of the webpage will be updated to display the website visitor count. Learn more about element.innerHTML from developer.mozilla.org/Element/innerHTML.
counterContainer.innerHTML = visitCount;
7. Implement Reset functionality triggered by button click
Now that visitor count is implemented, we need to add a reset button to allow users to reset the counter back to 1. The first step is to include the HTML code for the reset button and match the button using DOM querySelector() similar to “website-counter”.
The selected DOM element is attached with a click event listener to trigger the reset handler function. The website visitor count is reset by reinitializing visitCount to 1 and updating the value in localStorage. The visitor count is updated on the screen using element.innerHTML similar to previous step.
<button id="reset">Reset</button>
// Adding onClick event listener
resetButton.addEventListener("click", () => {
visitCount = 1;
localStorage.setItem("page_view", 1);
counterContainer.innerHTML = visitCount;
});
8. Add CSS Styles to website visitor counter
Once all the functionalities of the website visitor counter are implemented, it is now time to add CSS styles to the app in order to add a professional look and improve usability. The CSS file is then linked to the HTML webpage by using the <link> tag.
<link rel="stylesheet" href="styles.css">
Refer Final code section below for the styles.css code.
Final Code
index.html
<html>
<head>
<title>Website Counter</title>
<script defer src="index.js"></script>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div>Website visit count:</div>
<div class="website-counter"></div>
<button id="reset">Reset</button>
</body>
</html>
index.js
var counterContainer = document.querySelector(".website-counter");
var resetButton = document.querySelector("#reset");
var visitCount = localStorage.getItem("page_view");
// Check if page_view entry is present
if (visitCount) {
visitCount = Number(visitCount) + 1;
localStorage.setItem("page_view", visitCount);
} else {
visitCount = 1;
localStorage.setItem("page_view", 1);
}
counterContainer.innerHTML = visitCount;
// Adding onClick event listener
resetButton.addEventListener("click", () => {
visitCount = 1;
localStorage.setItem("page_view", 1);
counterContainer.innerHTML = visitCount;
});
style.css
/* Center child elements */
body,
.website-counter {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
body {
height: 100vh;
}
/* Styles for website counter container */
.website-counter {
background-color: #ff4957;
height: 50px;
width: 80px;
color: white;
border-radius: 30px;
font-weight: 700;
font-size: 25px;
margin-top: 10px;
}
/* Styles for reset button */
#reset {
margin-top: 20px;
background-color: #008cba;
cursor: pointer;
font-size: 18px;
padding: 8px 20px;
color: white;
border: 0;
}
Do you want to build an Amazing game in JavaScript? Read 6 Easy to build JavaScript Games for Beginners