In this article, we will discuss how to build an increment and decrement counter on button click. If you are considering only implementing increment functionality, then code for decrement can be ignored.
We will create a counter with the following functionalities:
- Initialize count to 0 on page load
- Increment count by 1 on button click
- Decrement count by 1 on button click
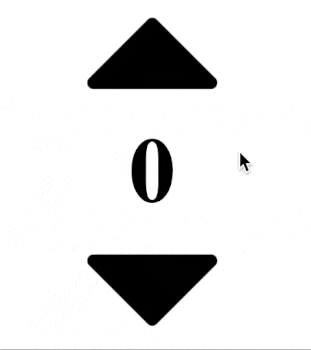
1. Create <div></div> for all the HTML elements
First, we need to HTML div tag for the following elements:
- Increment Button: HTML container for increment button with id “increment-count.
- Total count: HTML container where total count text is displayed.
- Decrement Button: HTML container for decrement button with id “decrement-count.
<div class="container">
<div id="increment-count">
</div>
<div id="total-count">
</div>
<div id="decrement-count">
</div>
</div>
2. Adding HTML Image Buttons
Next, we will add two buttons for both increment and decrement as follows:
- Image button with id as “up-arrow” and up_arrow.png image url as source.
- Image button with id as “down-arrow” and up_arrow.png image url as source.
<div class="container">
<div id="increment-count">
<input type="image" id="up-arrow" src="up_arrow.png" />
</div>
<div id="total-count">
</div>
<div id="decrement-count">
<input type="image" id="down-arrow" src="down_arrow.png" />
</div>
</div>
3. Initialize count to 0 on page load
The count needs to be displayed as 0 when the page is first loaded, this is accomplished by the following steps:
- Access the HTML container with id “total-count” using DOM selectors.
- Declare and initialize JS variable count with 0.
- Assign count variable value to previously selected DOM element innerHTML.
// Select total count
const totalCount = document.getElementById("total-count");
// Variable to track count
var count = 0;
// Display initial count value
totalCount.innerHTML = count;
4. JS function for Increment functionality
The JS function to handle count increment using the following steps:
- Increment count variable value by 1.
- Assign count variable value to totalCount DOM element.
// Function to increment count
const handleIncrement = () => {
count++;
totalCount.innerHTML = count;
};
5. JS function for Decrement functionality
The JS function to handle count decrement using the following steps:
- Decrement count variable value by 1.
- Assign count variable value to totalCount DOM element.
// Function to decrement count
const handleDecrement = () => {
count--;
totalCount.innerHTML = count;
};
6. Add “click” event to buttons
Finally, we need to allow users to trigger JavaScript functionality, which is implemented in the following steps:
- Access HTML Buttons from JavaScript using DOM selectors
- Add “click” event to both increment and decrement image buttons.
// Select increment and decrement buttons
const incrementCount = document.getElementById("increment-count");
const decrementCount = document.getElementById("decrement-count");
// Add click event to buttons
incrementCount.addEventListener("click", handleIncrement);
decrementCount.addEventListener("click", handleDecrement);
Check code for style.css below for CSS code for alignment and styles.
Final Solution Code
index.html
<html>
<head>
<title>Increment Decrement Counter</title>
<link rel="stylesheet" href="styles.css" />
<script src="main.js" defer></script>
</head>
<body>
<div class="container">
<div id="increment-count">
<input type="image" id="up-arrow" src="up_arrow.png" />
</div>
<div id="total-count">
</div>
<div id="decrement-count">
<input type="image" id="down-arrow" src="down_arrow.png" />
</div>
</div>
</body>
</html>
main.js
// Select increment and decrement buttons
const incrementCount = document.getElementById("increment-count");
const decrementCount = document.getElementById("decrement-count");
// Select total count
const totalCount = document.getElementById("total-count");
// Variable to track count
var count = 0;
// Display initial count value
totalCount.innerHTML = count;
// Function to increment count
const handleIncrement = () => {
count++;
totalCount.innerHTML = count;
};
// Function to decrement count
const handleDecrement = () => {
count--;
totalCount.innerHTML = count;
};
// Add click event to buttons
incrementCount.addEventListener("click", handleIncrement);
decrementCount.addEventListener("click", handleDecrement);
styles.css
#up-arrow,#down-arrow{
width: 100px;
height: 100px;
}
#total-count{
font-weight: 900;
font-size: 70px;
}
.container{
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
}