An image carousel displays a gallery of images and allows users to navigate with previous and next buttons. In this article, we will implement the following functionalities using vanilla javascript without any external libraries:
- Allow users to navigate between previous and next images.
- Automatically slideshow all slides with animation.
- Feature to pause image slideshow.
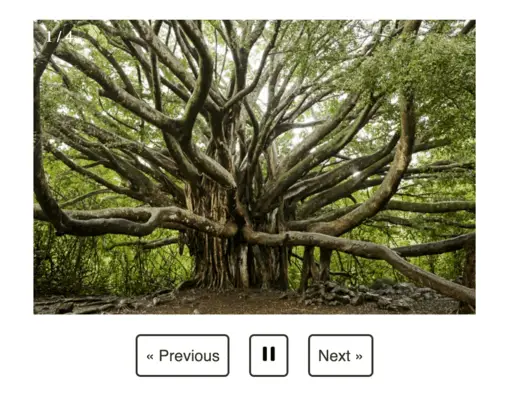
1) Load a list of images
The <div/> container for the carousel and parent <div/> for all the <img> tags is declared.
<div class="carousel">
<div class="image-label"></div>
<div class="image-gallery"></div>
</div>
The following JavaScript code ensures that the <img/> tag for every URL in the list is appended to the HTML document.
var imageList = [
"https://lh5.googleusercontent.com/xo6zDzj4Mq8JTuh31DRdzWPkmeekU1ykdvy7gmdGNkBnVzHoULgCA_MpL1ybOV2GKEkbvmswUl0iQW0lvnNQe3gqOFi_-bbt3MBzOAla29FvVN753jPZS87Bn7HyXoQ-dwA-ioYg",
"https://cdn.thomasnet.com/insights-images/eaf2ea91-c0ca-488d-ab63-af480b6f78cb/750px.png",
"https://moneyinc.com/wp-content/uploads/2018/11/Willow-750x500.jpg",
"https://japan.stripes.com/sites/default/files/styles/community_site_carousel_750x500/public/article-images/main_13.jpg?itok=_GELFbpY"
];
var imageGallery = document.querySelector(".image-gallery");
var imageLabel = document.querySelector(".image-label");
loadImage();
function loadImage() {
for (var url of imageList) {
imageGallery.innerHTML += `<img src="${url}"/>`;
}
}
2) Display the current image and hide inactive images
The following steps are required to add CSS styles to the carousel:
- Fixed width and height are specified for each image and the carousel container.
- “overflow: hidden” ensures that inactive images don’t overflow the carousel and are instead hidden.
- The <div/> with class name “image-gallery” is provided sufficient width to enclose all images. Additionally, the transition style is provided to support slide animation.
/* size of each Image */
.image-gallery img,
.carousel {
width: 450px;
height: 300px;
}
.carousel {
overflow: hidden; /* So the sliding bit doesn't stick out. */
margin: 0 auto;
}
/* Total size with animation */
.image-gallery {
width: 1800px;
-webkit-transition: all 1s ease-in-out;
-moz-transition: all 1s ease-in-out;
-o-transition: all 1s ease-in-out;
transition: all 1s ease-in-out;
}
3) Implement previous and next buttons
To implement the previous/next image feature, the current index must be incremented or decremented. Next, the image is transitioned from the current position to the position of the next image using CSS.
<div class="button-container">
<button id="prev-action">« Previous</button>
<button id="timer-action">
<img id="icon-img" />
</button>
<button id="next-action">Next »</button>
</div>
var currentIndex = 0;
var imageGallery = document.querySelector(".image-gallery");
var imageLabel = document.querySelector(".image-label");
function updateImage() {
imageGallery.style.transform = `translateX(-${position}px)`;
imageLabel.innerHTML = `${currentIndex + 1} / ${imageList.length}`;
}
function handleNext() {
currentIndex = (currentIndex + 1) % imageList.length;
updateImage();
}
function handlePrevious() {
currentIndex = currentIndex - 1;
updateImage();
}
4) Reset carousel before the first or after the last slide
The edge cases where the user clicks on the next button for the last image and the previous button for the first image must be handled. In handleNext() function when currentIndex refers to the last image of the gallery, the position of the next image is set to 0. Further, handlePrevious() for the first image, the destination position is set to the start point of the last image.
var position = 0;
function handleNext() {
if (currentIndex === imageList.length - 1) {
position = 0;
} else {
position = imageWidth * (currentIndex + 1);
}
currentIndex = (currentIndex + 1) % imageList.length;
updateImage();
}
function handlePrevious() {
currentIndex = currentIndex - 1;
if (currentIndex < 0) {
position = imageWidth * (imageList.length - 1);
currentIndex += imageList.length;
} else {
position = imageWidth * currentIndex;
}
updateImage();
}
5) Slideshow images every two seconds
The startSlideShow() function triggers the slideshow event for the carousel. The code “setInterval(handleNext, 2000)” ensures that handleNext() function is called every two seconds. As a result, the image slide transitions every two seconds.
var status;
var slideshow;
function startSlideShow() {
imageLabel.innerHTML = `${currentIndex + 1} / ${imageList.length}`;
slideshow = setInterval(handleNext, 2000);
iconImage.src = pauseIconUrl;
status = true;
}
To start slideshow on page load, startSlideShow() function is called after appending images to HTML file.
loadImage();
function loadImage() {
for (var url of imageList) {
imageGallery.innerHTML += `<img src="${url}"/>`;
}
startSlideShow();
}
6) Feature to Pause Slideshow
To stop the slideshow, the previous setinterval() action is reversed using the clearInterval() method.
function handlePause() {
clearInterval(slideshow);
iconImage.src = playIconUrl;
status = false;
}
The button action is toggled between the start and pause states.
function handleAction() {
if (status) {
handlePause();
} else {
startSlideShow();
}
}
document.getElementById("timer-action").addEventListener("click", handleAction);