In this article, we will learn how to show/hide an element based on their class names using JavaScript. Here are the following functionalities we will accomplish:
- Hide element by class name on button click
- Show hidden element by class name on button click
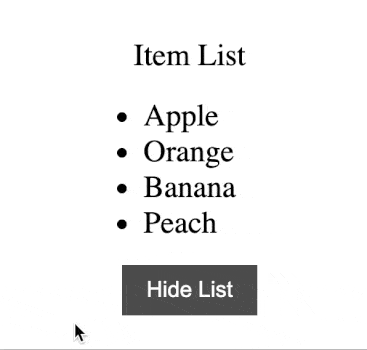
We will consider a webpage with an HTML unordered list of items. The hide and show functionality need to be added to a DOM element with the class name “item-list“.
<div class="title">Item List</div>
<div class="item-list">
<ul>
<li>Apple</li>
<li>Orange</li>
<li>Banana</li>
<li>Peach</li>
</ul>
</div>
1. Add button to show/hide the element
First, we need to add an HTML element to allow users to trigger show/hide functionality from the webpage. Hence, we will add an HTML button with the text “Hide List” as the element is displayed initially.
<button id="action">Hide List</button>
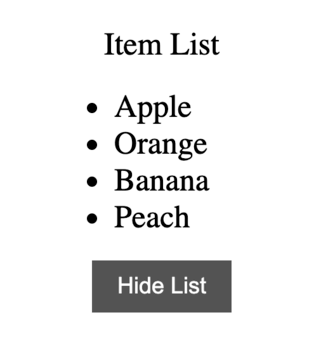
2. DOM selector to find the element by Class
To perform JS DOM manipulation, we need to first select the element using DOM selectors. There are two ways to select an element using a class which is as follows:
- document.querySelector() returns first element that satisfies the query.
- document.getElementsByClassName() return array of elements with the class name.
// Recommended approach
const actionButton = document.getElementById("action");
// Alternative approach
const actionButton = document.getElementsByClassName(".action")[0];
3. Show hidden element with display = “block”
We display a hidden element by assigning element.style.display with “block” and further the change text of the button to “Hide List” for indicating users that element can be hidden. Read more about element display from geeksforgeeks.org/html-dom-style-display-property/.
const toggleShowList = () => {
const isHidden = itemList.style.display === "none";
if (isHidden) {
// Display hidden element
itemList.style.display = "block";
actionButton.innerHTML = "Hide List";
}
};
4. Hide element with display = “none”
If the item list is already hidden then we display the element by element.style.display with “none”. Additionally, the text of the button is changed to “Show List“.
const toggleShowList = () => {
const isHidden = itemList.style.display === "none";
if (isHidden) {
itemList.style.display = "block";
actionButton.innerHTML = "Hide List";
} else {
// Hide element
itemList.style.display = "none";
actionButton.innerHTML = "Show List";
}
};
5. Attach “click” event listener for the button
To trigger the JavaScript code on the button click, we need to first select the button element using DOM selectors. Next, we will add event listeners to the selected DOM element. Hence, the toogleShowList function is triggered on the button click.
const actionButton = document.getElementById("action");
// Adding click event listener
actionButton.addEventListener("click", toggleShowList);
Final Solution Code
index.html
<!DOCTYPE html>
<html>
<head>
<title>Parcel Sandbox</title>
<meta charset="UTF-8" />
</head>
<body>
<div id="app">
<div class="title">Item List</div>
<div class="item-list">
<ul>
<li>Apple</li>
<li>Orange</li>
<li>Banana</li>
<li>Peach</li>
</ul>
</div>
<button id="action">Hide List</button>
</div>
<script src="main.js"></script>
</body>
</html>
main.js
import "./styles.css";
const actionButton = document.getElementById("action");
const itemList = document.querySelector(".item-list");
const toggleShowList = () => {
const isHidden = itemList.style.display === "none";
if (isHidden) {
// Display hidden element
itemList.style.display = "block";
actionButton.innerHTML = "Hide List";
} else {
// Hide element
itemList.style.display = "none";
actionButton.innerHTML = "Show List";
}
};
// Adding click event listener
actionButton.addEventListener("click", toggleShowList);
styles.css
* {
margin: 0;
padding: 0;
}
.title {
margin-bottom: 20px;
}
#app {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
font-size: 25px;
}
#action {
background-color: #555555;
border: none;
font-size: 18px;
margin-top: 20px;
color: #fff;
padding: 10px 20px;
cursor: pointer;
}