The CSV format is ideal for storing data in a file’s rows and columns. However, most data in web applications and unstructured databases like MongoDB are represented in JSON format. Hence, the data from CSV files need to be parsed in JSON format to be accessed and stored.
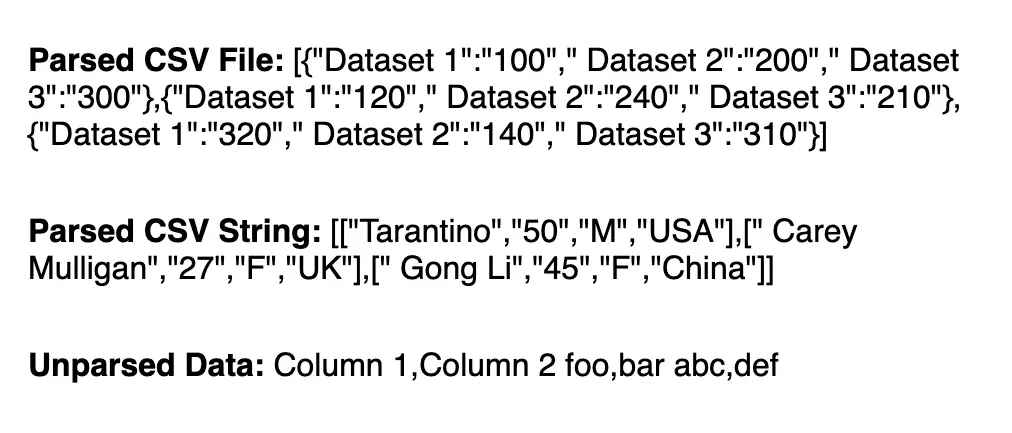
We will implement the following features:
- Parse String with delimited text into a JSON object.
- Download and parse remote CSV files into JSON format.
- Parse local CSV files into JSON format
- Unparse JSON object into CSV format with delimited text.
Install “papaparse” npm package
“papaparse” is an external npm package that provides functionalities to convert data from CSV to JSON and JSON to CSV formats. It can be installed from the terminal through the following commands:
npm i papaparse
// For projects configured with yarn
yarn add papaparse
Remote CSV file to a JSON object
First, react state is declared to store the data from the CSV file, and “,” is declared delimiter character.
const [CSVData, setCSVData] = useState();
var commonConfig = { delimiter: "," };
The Papa.parse() method will parse the remote CSV file when the URL of the file is passed as a parameter. Additionally, the “download” attribute of the config must be true, allowing the file to be downloaded before parsing.
The “complete” attribute requires a callback function which is triggered after parsing of the CSV file is successful.
Papa.parse(
"https://raw.githubusercontent.com/aniruddhaalek/web/master/chartsninja-data-1.csv",
{
...commonConfig,
header: true,
download: true,
complete: (result) => {
setCSVData(result.data);
}
}
);
Local CSV file to a JSON object
The HTML file input allows users to select a CSV file from the file system.
<input type="file" id="myfile" name="myfile">
The file element is accessed through a DOM selector and passed as a parameter for Papa.parse() function.
var fileInput = document.getElementById("myfile")
Papa.parse(
fileInput,
{
...commonConfig,
header: true,
complete: (result) => {
setCSVData(result.data);
}
}
);
Parsing CSV string to a JSON object
The CSV string with delimiter text when passed as the parameter is converted into a JSON object.
const [parsedString, setParsedString] = useState();
const CSVString =
"Tarantino,50,M,USA\n Carey Mulligan,27,F,UK\n Gong Li,45,F,China";
// Parse CSV String with delimiter text
Papa.parse(CSVString, {
...commonConfig,
complete: (result) => {
setParsedString(result.data);
}
});
Unparsing data from JSON to CSV format
The JSON object will be unparsed to CSV string with delimiter text using Papa.unparse() method.
const JSONObject = [
{
"Column 1": "foo",
"Column 2": "bar"
},
{
"Column 1": "abc",
"Column 2": "def"
}
];
setUnparsedString(Papa.unparse(JSONObject));
Final Solution Code
App.js
import Papa from "papaparse";
import React, { useState, useEffect } from "react";
import "./styles.css";
export default function App() {
var commonConfig = { delimiter: "," };
const [CSVData, setCSVData] = useState();
const [parsedString, setParsedString] = useState();
const [unparsedString, setUnparsedString] = useState();
const CSVString =
"Tarantino,50,M,USA\n Carey Mulligan,27,F,UK\n Gong Li,45,F,China";
const JSONObject = [
{
"Column 1": "foo",
"Column 2": "bar"
},
{
"Column 1": "abc",
"Column 2": "def"
}
];
// Parse remote CSV file
function parseCSVData() {
Papa.parse(
"https://raw.githubusercontent.com/aniruddhaalek/web/master/chartsninja-data-1.csv",
{
...commonConfig,
header: true,
download: true,
complete: (result) => {
setCSVData(result.data);
}
}
);
// Parse CSV String with delimiter text
Papa.parse(CSVString, {
...commonConfig,
complete: (result) => {
setParsedString(result.data);
}
});
// Unparse JSON Object into CSV format
setUnparsedString(Papa.unparse(JSONObject));
}
useEffect(() => {
parseCSVData();
}, []);
return (
<div className="main">
<div>
<b>Parsed CSV File:</b> {JSON.stringify(CSVFile)}
</div>
<div>
<b>Parsed CSV String:</b> {JSON.stringify(parsedString)}
</div>
<div>
<b>Unparsed Data:</b> {unparsedString}
</div>
</div>
);
}
styles.css
.main {
font-family: sans-serif;
height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 30px;
padding: 0px 30px;
}